Auto Pause Video Slider
using Vimeo
Video keeps playing with sound when you change slides? Adding this script will play video on the active slide and pause ones on the others.
Prerequisites
Vimeo Player.js
Vimeo's player.js is required for pause and play functionality.
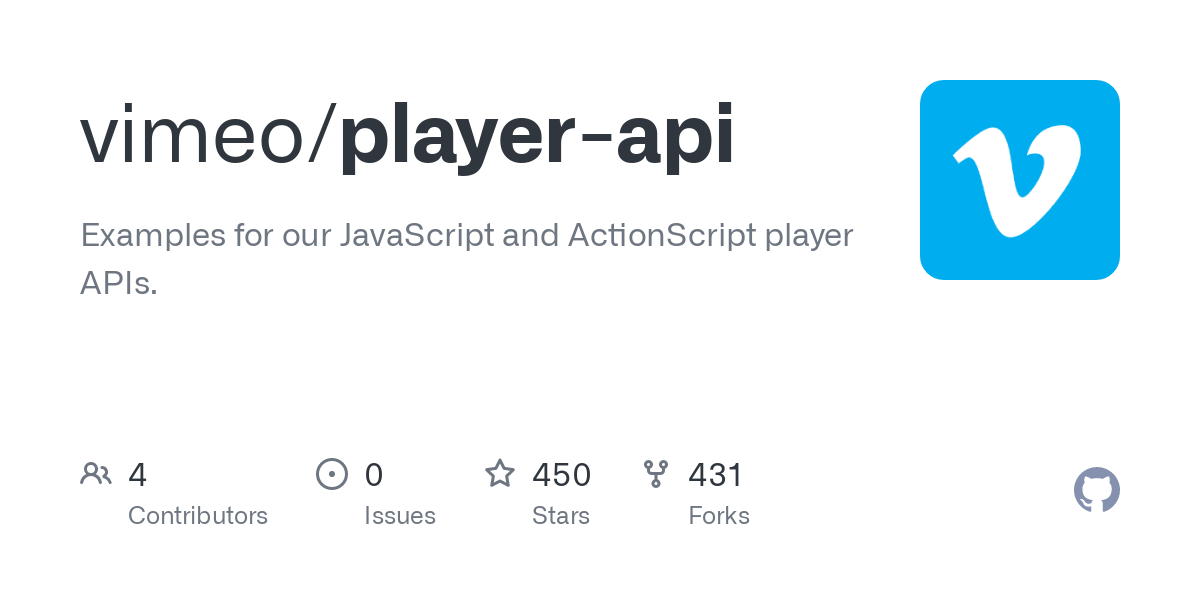
Video embed iFrame
Copy iFrame tag from Vimeo embed options:
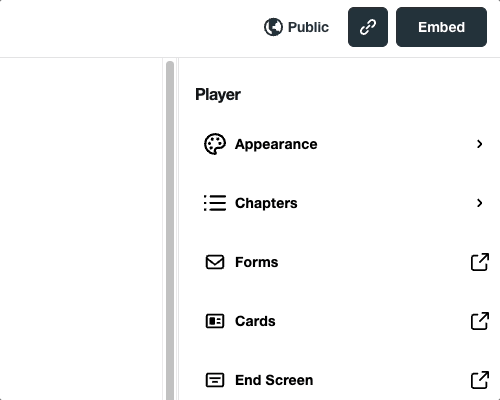
Use Cases
With Static Slider
Perfect when you know there are not going to be frequent updates to the videos.
Use Static SetupWith CMS Slider
Pixeto's Vimeo Slider combines with Finsweet's Dynamic Slider to deliver a CMS powered solution.
Use CMS SetupSetup
Vimeo's player.js is required for the Vimeo player to function.
1. Add this Vimeo Player.js CDN before closing /body tag
2. Add this Code before closing /body tag
How to use
There are two options in the calling function of the slider. You can change these options to select your Slider and Thumbnail elements.
slider:
Add slider=vimeo attribute to your Slider.
You can use class and ID as well:
Use '.your_slider_class' to select using class
Use '#your_slider_ID' to select using ID
thumbnail:
If using a custom thumbnail, replace with the class of your thumbnail element. (It can be a div, image or anything)
Script will hide this element when user clicks and start playing the video.
CMS Powered Vimeo Slider
CMS Setup

Copy numeric video id from the URL
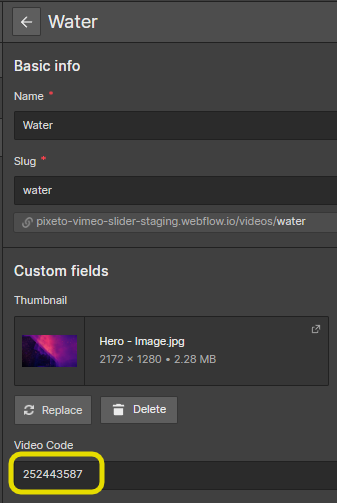
Create a CMS field and populate all of the IDs

Place newly created CMS field at the end of ".../video/" in the the iFrame
Setup
Vimeo's player.js is required for the Vimeo player to function.
1. Add this Vimeo Player.js CDN before closing /body tag
2. Add this Code before closing /body tag
How to use
There are two options in the calling function of the slider. You can change these options to select your Slider and Thumbnail elements.
slider:
Add slider=vimeo attribute to your Slider.
You can use class and ID as well:
Use '.your_slider_class' to select using class
Use '#your_slider_ID' to select using ID
thumbnail:
If using a custom thumbnail, replace with the class of your thumbnail element. (It can be a div, image or anything)
Script will hide this element when user clicks and start playing the video.